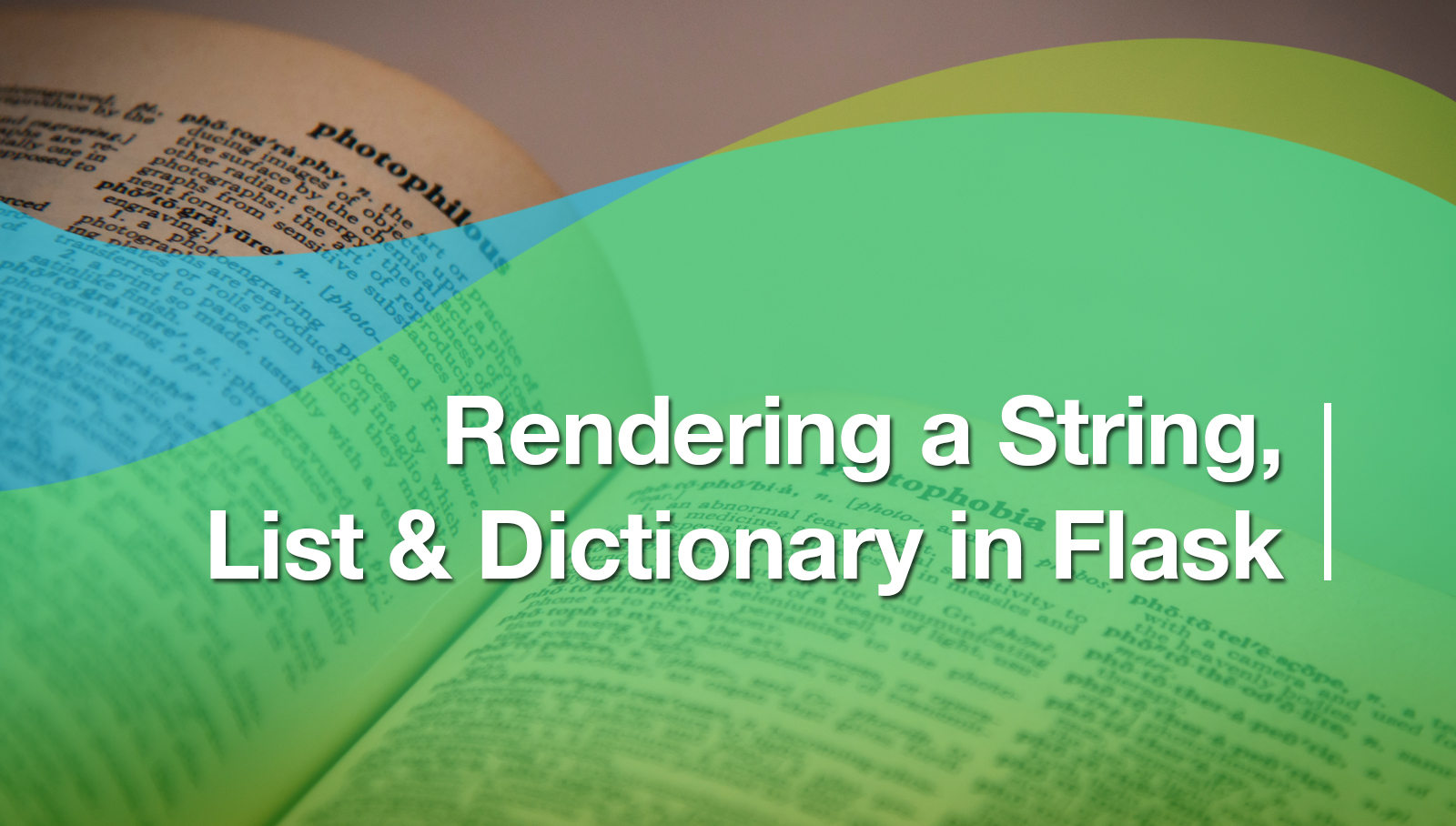
(Credit: Pugo Design Studio)
Flask is a lightweight, easy-to-use web framework for Python.
And what makes Flask great (to say, Django) is that it’s possible to build a web app using just a few lines of code. If you’re interested in learning how to use Flask, you can check out an awesome tutorial below.
Depending on your project, you may need to render a string, list, or dictionary in the HTML page from within a Flask app. When doing this, you’ll need to pass the variables from Python to the HTML, often using the render_template function within Flask.
For this, article, let’s use this sample code for rendering within the HTML of the Flask application.
#Python from flask import Flask, render_template app = Flask(__name__) def foo(): #Do something return bar # In this example, foo can return a string, list or dict @app.route('/') def index(): return render_template('index.html', foobar=bar) if __name__ == '__main__': app.run(debug=True)
Rendering a String
Within the HTML part of the app, you can use {{ foobar }} when you want to pass the contents of the foobar variable from the Python script to the HTML page.
In this case, foobar is a string.
<!DOCTYPE html> <html> <head> <title>Test App</title> </head> <body> {{ foobar }} #This returns the contents (string) stored in the `foobar` variable. </body> </html>
Rendering a List
When working with a list, things are a little different. Specifically, within the HTML, you need to iterate through the list and render each element on its own within the HTML.
In this case, foobar is a list.
<!DOCTYPE html> <html> <head> <title>Test App</title> </head> <body> {% for each in foobar %} {{ each }} {% end for %} </body> </html>
Rendering a Dictionary
Finally, when working with a dictionary, you need to iterate through the dictionary and render each element on its own.
In this case, foobar is a dictionary.
<!DOCTYPE html> <html> <head> <title>Test App</title> </head> <body> {% for key, value in foobar.items() %} {{ key }} : {{ value }} {% end for %} </body> </html>